When does the toUpperCase() method create a new object?
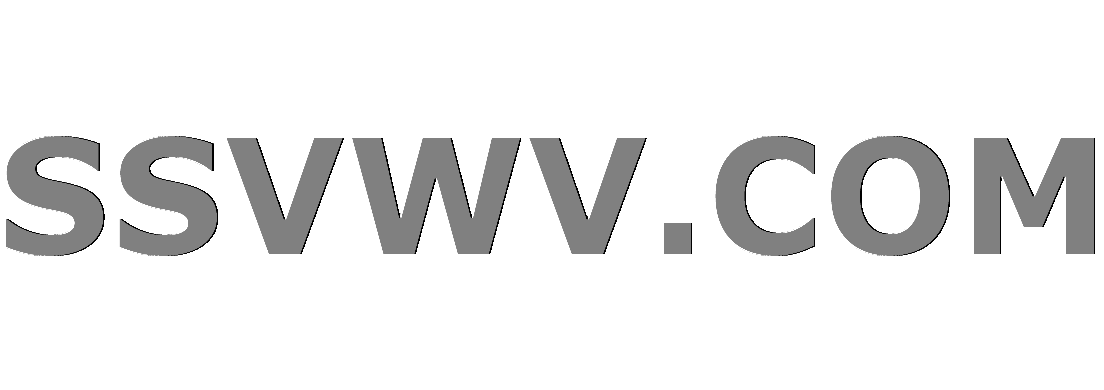
Multi tool use
up vote
11
down vote
favorite
public class Child{
public static void main(String args){
String x = new String("ABC");
String y = x.toUpperCase();
System.out.println(x == y);
}
}
Output: true
So does toUpperCase()
always create a new object?
java string object
add a comment |
up vote
11
down vote
favorite
public class Child{
public static void main(String args){
String x = new String("ABC");
String y = x.toUpperCase();
System.out.println(x == y);
}
}
Output: true
So does toUpperCase()
always create a new object?
java string object
2
I wouldn't rely on this behaviour but I would expect it to avoid creating a new object.
– Peter Lawrey
yesterday
Note: new String(...) doesn't change the answer.
– Peter Lawrey
yesterday
8
String x = new String("ABC"); Please don't do this. You create String object twice. just use x = "ABC";
– Sarief
yesterday
edit: someone pointed that OP used new String("ABC") to point to fact that it's not interned. I don't see how interning or not interning makes difference for toUpperCase(Locale) method
– Sarief
yesterday
add a comment |
up vote
11
down vote
favorite
up vote
11
down vote
favorite
public class Child{
public static void main(String args){
String x = new String("ABC");
String y = x.toUpperCase();
System.out.println(x == y);
}
}
Output: true
So does toUpperCase()
always create a new object?
java string object
public class Child{
public static void main(String args){
String x = new String("ABC");
String y = x.toUpperCase();
System.out.println(x == y);
}
}
Output: true
So does toUpperCase()
always create a new object?
java string object
java string object
edited yesterday
JJJ
29k147591
29k147591
asked yesterday
Rahul Dev
1589
1589
2
I wouldn't rely on this behaviour but I would expect it to avoid creating a new object.
– Peter Lawrey
yesterday
Note: new String(...) doesn't change the answer.
– Peter Lawrey
yesterday
8
String x = new String("ABC"); Please don't do this. You create String object twice. just use x = "ABC";
– Sarief
yesterday
edit: someone pointed that OP used new String("ABC") to point to fact that it's not interned. I don't see how interning or not interning makes difference for toUpperCase(Locale) method
– Sarief
yesterday
add a comment |
2
I wouldn't rely on this behaviour but I would expect it to avoid creating a new object.
– Peter Lawrey
yesterday
Note: new String(...) doesn't change the answer.
– Peter Lawrey
yesterday
8
String x = new String("ABC"); Please don't do this. You create String object twice. just use x = "ABC";
– Sarief
yesterday
edit: someone pointed that OP used new String("ABC") to point to fact that it's not interned. I don't see how interning or not interning makes difference for toUpperCase(Locale) method
– Sarief
yesterday
2
2
I wouldn't rely on this behaviour but I would expect it to avoid creating a new object.
– Peter Lawrey
yesterday
I wouldn't rely on this behaviour but I would expect it to avoid creating a new object.
– Peter Lawrey
yesterday
Note: new String(...) doesn't change the answer.
– Peter Lawrey
yesterday
Note: new String(...) doesn't change the answer.
– Peter Lawrey
yesterday
8
8
String x = new String("ABC"); Please don't do this. You create String object twice. just use x = "ABC";
– Sarief
yesterday
String x = new String("ABC"); Please don't do this. You create String object twice. just use x = "ABC";
– Sarief
yesterday
edit: someone pointed that OP used new String("ABC") to point to fact that it's not interned. I don't see how interning or not interning makes difference for toUpperCase(Locale) method
– Sarief
yesterday
edit: someone pointed that OP used new String("ABC") to point to fact that it's not interned. I don't see how interning or not interning makes difference for toUpperCase(Locale) method
– Sarief
yesterday
add a comment |
1 Answer
1
active
oldest
votes
up vote
16
down vote
accepted
toUpperCase()
calls toUpperCase(Locale.getDefault())
, which creates a new String
object only if it has to. If the input String
is already in upper case, it returns the input String
.
This seems to be an implementation detail, though. I didn't find it mentioned in the Javadoc.
Here's an implementation:
public String toUpperCase(Locale locale) {
if (locale == null) {
throw new NullPointerException();
}
int firstLower;
final int len = value.length;
/* Now check if there are any characters that need to be changed. */
scan: {
for (firstLower = 0 ; firstLower < len; ) {
int c = (int)value[firstLower];
int srcCount;
if ((c >= Character.MIN_HIGH_SURROGATE)
&& (c <= Character.MAX_HIGH_SURROGATE)) {
c = codePointAt(firstLower);
srcCount = Character.charCount(c);
} else {
srcCount = 1;
}
int upperCaseChar = Character.toUpperCaseEx(c);
if ((upperCaseChar == Character.ERROR)
|| (c != upperCaseChar)) {
break scan;
}
firstLower += srcCount;
}
return this; // <-- the original String is returned
}
....
}
4
@Sarief if it created a new object (and returned that new object)x == y
would definitely return false.
– Eran
yesterday
2
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
1
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
1
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string usingnew
and not invokedintern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question
– nits.kk
yesterday
2
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
|
show 7 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
16
down vote
accepted
toUpperCase()
calls toUpperCase(Locale.getDefault())
, which creates a new String
object only if it has to. If the input String
is already in upper case, it returns the input String
.
This seems to be an implementation detail, though. I didn't find it mentioned in the Javadoc.
Here's an implementation:
public String toUpperCase(Locale locale) {
if (locale == null) {
throw new NullPointerException();
}
int firstLower;
final int len = value.length;
/* Now check if there are any characters that need to be changed. */
scan: {
for (firstLower = 0 ; firstLower < len; ) {
int c = (int)value[firstLower];
int srcCount;
if ((c >= Character.MIN_HIGH_SURROGATE)
&& (c <= Character.MAX_HIGH_SURROGATE)) {
c = codePointAt(firstLower);
srcCount = Character.charCount(c);
} else {
srcCount = 1;
}
int upperCaseChar = Character.toUpperCaseEx(c);
if ((upperCaseChar == Character.ERROR)
|| (c != upperCaseChar)) {
break scan;
}
firstLower += srcCount;
}
return this; // <-- the original String is returned
}
....
}
4
@Sarief if it created a new object (and returned that new object)x == y
would definitely return false.
– Eran
yesterday
2
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
1
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
1
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string usingnew
and not invokedintern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question
– nits.kk
yesterday
2
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
|
show 7 more comments
up vote
16
down vote
accepted
toUpperCase()
calls toUpperCase(Locale.getDefault())
, which creates a new String
object only if it has to. If the input String
is already in upper case, it returns the input String
.
This seems to be an implementation detail, though. I didn't find it mentioned in the Javadoc.
Here's an implementation:
public String toUpperCase(Locale locale) {
if (locale == null) {
throw new NullPointerException();
}
int firstLower;
final int len = value.length;
/* Now check if there are any characters that need to be changed. */
scan: {
for (firstLower = 0 ; firstLower < len; ) {
int c = (int)value[firstLower];
int srcCount;
if ((c >= Character.MIN_HIGH_SURROGATE)
&& (c <= Character.MAX_HIGH_SURROGATE)) {
c = codePointAt(firstLower);
srcCount = Character.charCount(c);
} else {
srcCount = 1;
}
int upperCaseChar = Character.toUpperCaseEx(c);
if ((upperCaseChar == Character.ERROR)
|| (c != upperCaseChar)) {
break scan;
}
firstLower += srcCount;
}
return this; // <-- the original String is returned
}
....
}
4
@Sarief if it created a new object (and returned that new object)x == y
would definitely return false.
– Eran
yesterday
2
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
1
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
1
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string usingnew
and not invokedintern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question
– nits.kk
yesterday
2
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
|
show 7 more comments
up vote
16
down vote
accepted
up vote
16
down vote
accepted
toUpperCase()
calls toUpperCase(Locale.getDefault())
, which creates a new String
object only if it has to. If the input String
is already in upper case, it returns the input String
.
This seems to be an implementation detail, though. I didn't find it mentioned in the Javadoc.
Here's an implementation:
public String toUpperCase(Locale locale) {
if (locale == null) {
throw new NullPointerException();
}
int firstLower;
final int len = value.length;
/* Now check if there are any characters that need to be changed. */
scan: {
for (firstLower = 0 ; firstLower < len; ) {
int c = (int)value[firstLower];
int srcCount;
if ((c >= Character.MIN_HIGH_SURROGATE)
&& (c <= Character.MAX_HIGH_SURROGATE)) {
c = codePointAt(firstLower);
srcCount = Character.charCount(c);
} else {
srcCount = 1;
}
int upperCaseChar = Character.toUpperCaseEx(c);
if ((upperCaseChar == Character.ERROR)
|| (c != upperCaseChar)) {
break scan;
}
firstLower += srcCount;
}
return this; // <-- the original String is returned
}
....
}
toUpperCase()
calls toUpperCase(Locale.getDefault())
, which creates a new String
object only if it has to. If the input String
is already in upper case, it returns the input String
.
This seems to be an implementation detail, though. I didn't find it mentioned in the Javadoc.
Here's an implementation:
public String toUpperCase(Locale locale) {
if (locale == null) {
throw new NullPointerException();
}
int firstLower;
final int len = value.length;
/* Now check if there are any characters that need to be changed. */
scan: {
for (firstLower = 0 ; firstLower < len; ) {
int c = (int)value[firstLower];
int srcCount;
if ((c >= Character.MIN_HIGH_SURROGATE)
&& (c <= Character.MAX_HIGH_SURROGATE)) {
c = codePointAt(firstLower);
srcCount = Character.charCount(c);
} else {
srcCount = 1;
}
int upperCaseChar = Character.toUpperCaseEx(c);
if ((upperCaseChar == Character.ERROR)
|| (c != upperCaseChar)) {
break scan;
}
firstLower += srcCount;
}
return this; // <-- the original String is returned
}
....
}
edited yesterday
answered yesterday


Eran
272k35431514
272k35431514
4
@Sarief if it created a new object (and returned that new object)x == y
would definitely return false.
– Eran
yesterday
2
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
1
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
1
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string usingnew
and not invokedintern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question
– nits.kk
yesterday
2
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
|
show 7 more comments
4
@Sarief if it created a new object (and returned that new object)x == y
would definitely return false.
– Eran
yesterday
2
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
1
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
1
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string usingnew
and not invokedintern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question
– nits.kk
yesterday
2
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
4
4
@Sarief if it created a new object (and returned that new object)
x == y
would definitely return false.– Eran
yesterday
@Sarief if it created a new object (and returned that new object)
x == y
would definitely return false.– Eran
yesterday
2
2
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
@Sarief it somehow got to this list, which tends to result in high traffic.
– Eran
yesterday
1
1
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
@Eran Let me rephrase, even if it had in contract that it creates a new object and it did create a new object, it does not mean that it will return a new object. Strings are contained in Strings pool and are reused mostly from there
– Sarief
yesterday
1
1
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string using
new
and not invoked intern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question– nits.kk
yesterday
@Sarief First of all its not silly question, as Strings are immutable so any operation performed is expected to result in a separate object. Secondly the answer as many would think is related to same string in string pool, but its not as OP has created string using
new
and not invoked intern()
hence correctly the answer points the implementation bwing the result as why no new object was created for the case presented by the question– nits.kk
yesterday
2
2
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
@nits.kk this should not apply for immutable objects, which Strings are
– Sarief
yesterday
|
show 7 more comments
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53370200%2fwhen-does-the-touppercase-method-create-a-new-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Y2x7,lxK,CC,O,Y8DcMc Qx0XxLN
2
I wouldn't rely on this behaviour but I would expect it to avoid creating a new object.
– Peter Lawrey
yesterday
Note: new String(...) doesn't change the answer.
– Peter Lawrey
yesterday
8
String x = new String("ABC"); Please don't do this. You create String object twice. just use x = "ABC";
– Sarief
yesterday
edit: someone pointed that OP used new String("ABC") to point to fact that it's not interned. I don't see how interning or not interning makes difference for toUpperCase(Locale) method
– Sarief
yesterday