bash: expecting number addition with +=. Not exactly sure if the operand is a number
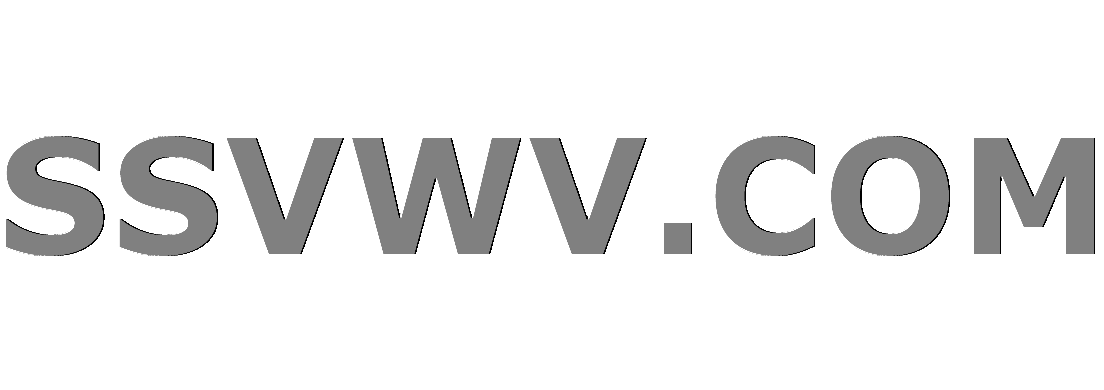
Multi tool use
up vote
2
down vote
favorite
I am trying to detect armstrong numbers with this code:
declare -i INPUT=$1
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$((${arr[index]}**${#arr[@]}))
done
echo "$armstrong_sum"
Commands to run the code: ./armstrong_sum 9
, armstrong_sum 10
and ./armstrong_sum 153
Output: 9
, 10
and 112527
Expected output: 9
, 1
and 153
An armstrong number is a number that is the sum of its own digits each raised to the power of number of digits
More importantly I want to be able to debug the script myself. Not exactly sure how one debugs in bash. Like get type of operand and see pause iteration at each step
bash
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
2
down vote
favorite
I am trying to detect armstrong numbers with this code:
declare -i INPUT=$1
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$((${arr[index]}**${#arr[@]}))
done
echo "$armstrong_sum"
Commands to run the code: ./armstrong_sum 9
, armstrong_sum 10
and ./armstrong_sum 153
Output: 9
, 10
and 112527
Expected output: 9
, 1
and 153
An armstrong number is a number that is the sum of its own digits each raised to the power of number of digits
More importantly I want to be able to debug the script myself. Not exactly sure how one debugs in bash. Like get type of operand and see pause iteration at each step
bash
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
your expected output doesnt make sense - by your own definition 153 should return 729
– Steven Penny
2 days ago
1^3 + 5^3 + 3^3 = 153
. This my expected output, i.e an armstrong number
– HarshvardhanSharma
2 days ago
Your second question (the bold part) already has an answer, easily found by searching: unix.stackexchange.com/questions/155551/…
– JigglyNaga
2 days ago
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am trying to detect armstrong numbers with this code:
declare -i INPUT=$1
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$((${arr[index]}**${#arr[@]}))
done
echo "$armstrong_sum"
Commands to run the code: ./armstrong_sum 9
, armstrong_sum 10
and ./armstrong_sum 153
Output: 9
, 10
and 112527
Expected output: 9
, 1
and 153
An armstrong number is a number that is the sum of its own digits each raised to the power of number of digits
More importantly I want to be able to debug the script myself. Not exactly sure how one debugs in bash. Like get type of operand and see pause iteration at each step
bash
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I am trying to detect armstrong numbers with this code:
declare -i INPUT=$1
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$((${arr[index]}**${#arr[@]}))
done
echo "$armstrong_sum"
Commands to run the code: ./armstrong_sum 9
, armstrong_sum 10
and ./armstrong_sum 153
Output: 9
, 10
and 112527
Expected output: 9
, 1
and 153
An armstrong number is a number that is the sum of its own digits each raised to the power of number of digits
More importantly I want to be able to debug the script myself. Not exactly sure how one debugs in bash. Like get type of operand and see pause iteration at each step
bash
bash
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 2 days ago
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Dec 7 at 3:30
HarshvardhanSharma
316
316
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
HarshvardhanSharma is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
your expected output doesnt make sense - by your own definition 153 should return 729
– Steven Penny
2 days ago
1^3 + 5^3 + 3^3 = 153
. This my expected output, i.e an armstrong number
– HarshvardhanSharma
2 days ago
Your second question (the bold part) already has an answer, easily found by searching: unix.stackexchange.com/questions/155551/…
– JigglyNaga
2 days ago
add a comment |
your expected output doesnt make sense - by your own definition 153 should return 729
– Steven Penny
2 days ago
1^3 + 5^3 + 3^3 = 153
. This my expected output, i.e an armstrong number
– HarshvardhanSharma
2 days ago
Your second question (the bold part) already has an answer, easily found by searching: unix.stackexchange.com/questions/155551/…
– JigglyNaga
2 days ago
your expected output doesnt make sense - by your own definition 153 should return 729
– Steven Penny
2 days ago
your expected output doesnt make sense - by your own definition 153 should return 729
– Steven Penny
2 days ago
1^3 + 5^3 + 3^3 = 153
. This my expected output, i.e an armstrong number– HarshvardhanSharma
2 days ago
1^3 + 5^3 + 3^3 = 153
. This my expected output, i.e an armstrong number– HarshvardhanSharma
2 days ago
Your second question (the bold part) already has an answer, easily found by searching: unix.stackexchange.com/questions/155551/…
– JigglyNaga
2 days ago
Your second question (the bold part) already has an answer, easily found by searching: unix.stackexchange.com/questions/155551/…
– JigglyNaga
2 days ago
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
As noted in man bash
(emphasis mine)
When += is applied to a variable for which the
integer attribute has been set, value is evaluated as an arithmetic
expression and added to the variable's current value, which is also
evaluated. When += is applied to an array variable using compound
assignment (see Arrays below), the variable's value is not unset (as it
is when using =), and new values are appended to the array beginning at
one greater than the array's maximum index (for indexed arrays) or
added as additional key-value pairs in an associative array. When
applied to a string-valued variable, value is expanded and appended to
the variable's value.
You are clearly getting the latter, i.e.
1 + 125 + 27 = 112527
So you have a couple of options - either declare armstrong_sum
as integer
#!/bin/bash
declare -i INPUT=$1
declare -i armstrong_sum=0
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$(( ${arr[index]}**${#arr[@]} ))
done
echo "$armstrong_sum"
or ensure arithmetic evaluation by surrounding the whole expression in ((
and ))
i.e.
(( armstrong_sum += ${arr[index]}**${#arr[@]} ))
add a comment |
up vote
0
down vote
Use https://www.shellcheck.net (I use it as syntactic vim plugin making a crude IDE)
I'd go with this;
#!/bin/bash
P="$(echo -n "$1" | wc -c)"
SUM=0;
for X in $(echo "$1" | fold -w 1) ; do
SUM=$(echo "$SUM+($X^$P)" | bc );
done
echo "$SUM"
It's not "pure" bash but I find the power of bash is the wide selection of tools, and prioritizing legibility.
for stack traces if you add the following to the top of all your scripts it will inform you of errors;
set -e
trap 'echo "ERROR: $BASH_SOURCE:$LINENO $BASH_COMMAND" >&2' ERR
it will stop the script on the error line, make output like
test.sh: line 7: no: command not found
ERROR: test.sh:7 no + 5
instead of (potentially silently) ignoring errors.
Use -x for debugging;
bash -x armstrong.sh 222
++ echo -n 222
++ wc -c
+ P=3
+ SUM=0
++ fold -w 1
++ echo 222
+ for X in $(echo "$1" | fold -w 1)
++ echo '0+(2^3)'
++ bc
+ SUM=8
+ for X in $(echo "$1" | fold -w 1)
++ echo '8+(2^3)'
++ bc
+ SUM=16
+ for X in $(echo "$1" | fold -w 1)
++ echo '16+(2^3)'
++ bc
+ SUM=24
+ echo 24
24
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
As noted in man bash
(emphasis mine)
When += is applied to a variable for which the
integer attribute has been set, value is evaluated as an arithmetic
expression and added to the variable's current value, which is also
evaluated. When += is applied to an array variable using compound
assignment (see Arrays below), the variable's value is not unset (as it
is when using =), and new values are appended to the array beginning at
one greater than the array's maximum index (for indexed arrays) or
added as additional key-value pairs in an associative array. When
applied to a string-valued variable, value is expanded and appended to
the variable's value.
You are clearly getting the latter, i.e.
1 + 125 + 27 = 112527
So you have a couple of options - either declare armstrong_sum
as integer
#!/bin/bash
declare -i INPUT=$1
declare -i armstrong_sum=0
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$(( ${arr[index]}**${#arr[@]} ))
done
echo "$armstrong_sum"
or ensure arithmetic evaluation by surrounding the whole expression in ((
and ))
i.e.
(( armstrong_sum += ${arr[index]}**${#arr[@]} ))
add a comment |
up vote
2
down vote
accepted
As noted in man bash
(emphasis mine)
When += is applied to a variable for which the
integer attribute has been set, value is evaluated as an arithmetic
expression and added to the variable's current value, which is also
evaluated. When += is applied to an array variable using compound
assignment (see Arrays below), the variable's value is not unset (as it
is when using =), and new values are appended to the array beginning at
one greater than the array's maximum index (for indexed arrays) or
added as additional key-value pairs in an associative array. When
applied to a string-valued variable, value is expanded and appended to
the variable's value.
You are clearly getting the latter, i.e.
1 + 125 + 27 = 112527
So you have a couple of options - either declare armstrong_sum
as integer
#!/bin/bash
declare -i INPUT=$1
declare -i armstrong_sum=0
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$(( ${arr[index]}**${#arr[@]} ))
done
echo "$armstrong_sum"
or ensure arithmetic evaluation by surrounding the whole expression in ((
and ))
i.e.
(( armstrong_sum += ${arr[index]}**${#arr[@]} ))
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
As noted in man bash
(emphasis mine)
When += is applied to a variable for which the
integer attribute has been set, value is evaluated as an arithmetic
expression and added to the variable's current value, which is also
evaluated. When += is applied to an array variable using compound
assignment (see Arrays below), the variable's value is not unset (as it
is when using =), and new values are appended to the array beginning at
one greater than the array's maximum index (for indexed arrays) or
added as additional key-value pairs in an associative array. When
applied to a string-valued variable, value is expanded and appended to
the variable's value.
You are clearly getting the latter, i.e.
1 + 125 + 27 = 112527
So you have a couple of options - either declare armstrong_sum
as integer
#!/bin/bash
declare -i INPUT=$1
declare -i armstrong_sum=0
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$(( ${arr[index]}**${#arr[@]} ))
done
echo "$armstrong_sum"
or ensure arithmetic evaluation by surrounding the whole expression in ((
and ))
i.e.
(( armstrong_sum += ${arr[index]}**${#arr[@]} ))
As noted in man bash
(emphasis mine)
When += is applied to a variable for which the
integer attribute has been set, value is evaluated as an arithmetic
expression and added to the variable's current value, which is also
evaluated. When += is applied to an array variable using compound
assignment (see Arrays below), the variable's value is not unset (as it
is when using =), and new values are appended to the array beginning at
one greater than the array's maximum index (for indexed arrays) or
added as additional key-value pairs in an associative array. When
applied to a string-valued variable, value is expanded and appended to
the variable's value.
You are clearly getting the latter, i.e.
1 + 125 + 27 = 112527
So you have a couple of options - either declare armstrong_sum
as integer
#!/bin/bash
declare -i INPUT=$1
declare -i armstrong_sum=0
arr=($(fold -w1 <<< "$INPUT"))
for index in "${!arr[@]}"
do
armstrong_sum+=$(( ${arr[index]}**${#arr[@]} ))
done
echo "$armstrong_sum"
or ensure arithmetic evaluation by surrounding the whole expression in ((
and ))
i.e.
(( armstrong_sum += ${arr[index]}**${#arr[@]} ))
answered 2 days ago
steeldriver
33.9k34983
33.9k34983
add a comment |
add a comment |
up vote
0
down vote
Use https://www.shellcheck.net (I use it as syntactic vim plugin making a crude IDE)
I'd go with this;
#!/bin/bash
P="$(echo -n "$1" | wc -c)"
SUM=0;
for X in $(echo "$1" | fold -w 1) ; do
SUM=$(echo "$SUM+($X^$P)" | bc );
done
echo "$SUM"
It's not "pure" bash but I find the power of bash is the wide selection of tools, and prioritizing legibility.
for stack traces if you add the following to the top of all your scripts it will inform you of errors;
set -e
trap 'echo "ERROR: $BASH_SOURCE:$LINENO $BASH_COMMAND" >&2' ERR
it will stop the script on the error line, make output like
test.sh: line 7: no: command not found
ERROR: test.sh:7 no + 5
instead of (potentially silently) ignoring errors.
Use -x for debugging;
bash -x armstrong.sh 222
++ echo -n 222
++ wc -c
+ P=3
+ SUM=0
++ fold -w 1
++ echo 222
+ for X in $(echo "$1" | fold -w 1)
++ echo '0+(2^3)'
++ bc
+ SUM=8
+ for X in $(echo "$1" | fold -w 1)
++ echo '8+(2^3)'
++ bc
+ SUM=16
+ for X in $(echo "$1" | fold -w 1)
++ echo '16+(2^3)'
++ bc
+ SUM=24
+ echo 24
24
add a comment |
up vote
0
down vote
Use https://www.shellcheck.net (I use it as syntactic vim plugin making a crude IDE)
I'd go with this;
#!/bin/bash
P="$(echo -n "$1" | wc -c)"
SUM=0;
for X in $(echo "$1" | fold -w 1) ; do
SUM=$(echo "$SUM+($X^$P)" | bc );
done
echo "$SUM"
It's not "pure" bash but I find the power of bash is the wide selection of tools, and prioritizing legibility.
for stack traces if you add the following to the top of all your scripts it will inform you of errors;
set -e
trap 'echo "ERROR: $BASH_SOURCE:$LINENO $BASH_COMMAND" >&2' ERR
it will stop the script on the error line, make output like
test.sh: line 7: no: command not found
ERROR: test.sh:7 no + 5
instead of (potentially silently) ignoring errors.
Use -x for debugging;
bash -x armstrong.sh 222
++ echo -n 222
++ wc -c
+ P=3
+ SUM=0
++ fold -w 1
++ echo 222
+ for X in $(echo "$1" | fold -w 1)
++ echo '0+(2^3)'
++ bc
+ SUM=8
+ for X in $(echo "$1" | fold -w 1)
++ echo '8+(2^3)'
++ bc
+ SUM=16
+ for X in $(echo "$1" | fold -w 1)
++ echo '16+(2^3)'
++ bc
+ SUM=24
+ echo 24
24
add a comment |
up vote
0
down vote
up vote
0
down vote
Use https://www.shellcheck.net (I use it as syntactic vim plugin making a crude IDE)
I'd go with this;
#!/bin/bash
P="$(echo -n "$1" | wc -c)"
SUM=0;
for X in $(echo "$1" | fold -w 1) ; do
SUM=$(echo "$SUM+($X^$P)" | bc );
done
echo "$SUM"
It's not "pure" bash but I find the power of bash is the wide selection of tools, and prioritizing legibility.
for stack traces if you add the following to the top of all your scripts it will inform you of errors;
set -e
trap 'echo "ERROR: $BASH_SOURCE:$LINENO $BASH_COMMAND" >&2' ERR
it will stop the script on the error line, make output like
test.sh: line 7: no: command not found
ERROR: test.sh:7 no + 5
instead of (potentially silently) ignoring errors.
Use -x for debugging;
bash -x armstrong.sh 222
++ echo -n 222
++ wc -c
+ P=3
+ SUM=0
++ fold -w 1
++ echo 222
+ for X in $(echo "$1" | fold -w 1)
++ echo '0+(2^3)'
++ bc
+ SUM=8
+ for X in $(echo "$1" | fold -w 1)
++ echo '8+(2^3)'
++ bc
+ SUM=16
+ for X in $(echo "$1" | fold -w 1)
++ echo '16+(2^3)'
++ bc
+ SUM=24
+ echo 24
24
Use https://www.shellcheck.net (I use it as syntactic vim plugin making a crude IDE)
I'd go with this;
#!/bin/bash
P="$(echo -n "$1" | wc -c)"
SUM=0;
for X in $(echo "$1" | fold -w 1) ; do
SUM=$(echo "$SUM+($X^$P)" | bc );
done
echo "$SUM"
It's not "pure" bash but I find the power of bash is the wide selection of tools, and prioritizing legibility.
for stack traces if you add the following to the top of all your scripts it will inform you of errors;
set -e
trap 'echo "ERROR: $BASH_SOURCE:$LINENO $BASH_COMMAND" >&2' ERR
it will stop the script on the error line, make output like
test.sh: line 7: no: command not found
ERROR: test.sh:7 no + 5
instead of (potentially silently) ignoring errors.
Use -x for debugging;
bash -x armstrong.sh 222
++ echo -n 222
++ wc -c
+ P=3
+ SUM=0
++ fold -w 1
++ echo 222
+ for X in $(echo "$1" | fold -w 1)
++ echo '0+(2^3)'
++ bc
+ SUM=8
+ for X in $(echo "$1" | fold -w 1)
++ echo '8+(2^3)'
++ bc
+ SUM=16
+ for X in $(echo "$1" | fold -w 1)
++ echo '16+(2^3)'
++ bc
+ SUM=24
+ echo 24
24
edited 2 days ago
answered 2 days ago
user1133275
2,750415
2,750415
add a comment |
add a comment |
HarshvardhanSharma is a new contributor. Be nice, and check out our Code of Conduct.
HarshvardhanSharma is a new contributor. Be nice, and check out our Code of Conduct.
HarshvardhanSharma is a new contributor. Be nice, and check out our Code of Conduct.
HarshvardhanSharma is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f486490%2fbash-expecting-number-addition-with-not-exactly-sure-if-the-operand-is-a-nu%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S7dNfQ z,K
your expected output doesnt make sense - by your own definition 153 should return 729
– Steven Penny
2 days ago
1^3 + 5^3 + 3^3 = 153
. This my expected output, i.e an armstrong number– HarshvardhanSharma
2 days ago
Your second question (the bold part) already has an answer, easily found by searching: unix.stackexchange.com/questions/155551/…
– JigglyNaga
2 days ago