C++ NULL vs __null
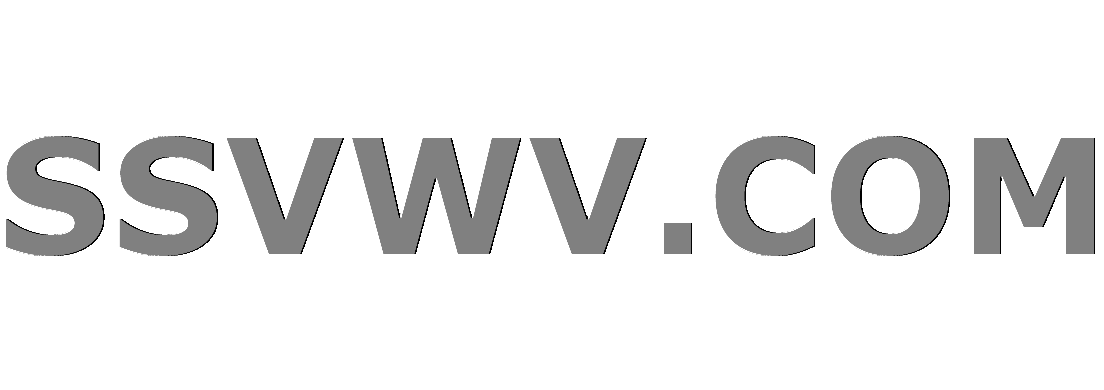
Multi tool use
I have the following code:
MyType x = NULL;
NetBeans gave me a suggestion to change it to this:
MyType x = __null;
I looked it up and found that __null
is called a "compiler keyword", which I assumed to mean it's used internally for the compiler. I don't understand why NetBeans suggested to change it to a compiler keyword.
What's the difference between NULL
and __null
in c++?
c++ keyword
add a comment |
I have the following code:
MyType x = NULL;
NetBeans gave me a suggestion to change it to this:
MyType x = __null;
I looked it up and found that __null
is called a "compiler keyword", which I assumed to mean it's used internally for the compiler. I don't understand why NetBeans suggested to change it to a compiler keyword.
What's the difference between NULL
and __null
in c++?
c++ keyword
14
In C++11 and after you should use the keyword nullptr.
– Anon Mail
1 hour ago
1
Don't use__null
. If it's an implementation detail, it's not portable to use it. If it's defined by the project, it's using a name reserved for use by the implementation, it's not legal to use that identifier and it should be removed from the project.
– François Andrieux
1 hour ago
If you wantNULL
usenullptr
. If that doesn't compile then you don't have a pointer and you are doing the wrong thing.
– NathanOliver
1 hour ago
@Some That's probably an appropriate answer to the question.
– πάντα ῥεῖ
1 hour ago
You wantnullptr
- always.
– Jesper Juhl
1 hour ago
add a comment |
I have the following code:
MyType x = NULL;
NetBeans gave me a suggestion to change it to this:
MyType x = __null;
I looked it up and found that __null
is called a "compiler keyword", which I assumed to mean it's used internally for the compiler. I don't understand why NetBeans suggested to change it to a compiler keyword.
What's the difference between NULL
and __null
in c++?
c++ keyword
I have the following code:
MyType x = NULL;
NetBeans gave me a suggestion to change it to this:
MyType x = __null;
I looked it up and found that __null
is called a "compiler keyword", which I assumed to mean it's used internally for the compiler. I don't understand why NetBeans suggested to change it to a compiler keyword.
What's the difference between NULL
and __null
in c++?
c++ keyword
c++ keyword
asked 1 hour ago


David the third
1,2461721
1,2461721
14
In C++11 and after you should use the keyword nullptr.
– Anon Mail
1 hour ago
1
Don't use__null
. If it's an implementation detail, it's not portable to use it. If it's defined by the project, it's using a name reserved for use by the implementation, it's not legal to use that identifier and it should be removed from the project.
– François Andrieux
1 hour ago
If you wantNULL
usenullptr
. If that doesn't compile then you don't have a pointer and you are doing the wrong thing.
– NathanOliver
1 hour ago
@Some That's probably an appropriate answer to the question.
– πάντα ῥεῖ
1 hour ago
You wantnullptr
- always.
– Jesper Juhl
1 hour ago
add a comment |
14
In C++11 and after you should use the keyword nullptr.
– Anon Mail
1 hour ago
1
Don't use__null
. If it's an implementation detail, it's not portable to use it. If it's defined by the project, it's using a name reserved for use by the implementation, it's not legal to use that identifier and it should be removed from the project.
– François Andrieux
1 hour ago
If you wantNULL
usenullptr
. If that doesn't compile then you don't have a pointer and you are doing the wrong thing.
– NathanOliver
1 hour ago
@Some That's probably an appropriate answer to the question.
– πάντα ῥεῖ
1 hour ago
You wantnullptr
- always.
– Jesper Juhl
1 hour ago
14
14
In C++11 and after you should use the keyword nullptr.
– Anon Mail
1 hour ago
In C++11 and after you should use the keyword nullptr.
– Anon Mail
1 hour ago
1
1
Don't use
__null
. If it's an implementation detail, it's not portable to use it. If it's defined by the project, it's using a name reserved for use by the implementation, it's not legal to use that identifier and it should be removed from the project.– François Andrieux
1 hour ago
Don't use
__null
. If it's an implementation detail, it's not portable to use it. If it's defined by the project, it's using a name reserved for use by the implementation, it's not legal to use that identifier and it should be removed from the project.– François Andrieux
1 hour ago
If you want
NULL
use nullptr
. If that doesn't compile then you don't have a pointer and you are doing the wrong thing.– NathanOliver
1 hour ago
If you want
NULL
use nullptr
. If that doesn't compile then you don't have a pointer and you are doing the wrong thing.– NathanOliver
1 hour ago
@Some That's probably an appropriate answer to the question.
– πάντα ῥεῖ
1 hour ago
@Some That's probably an appropriate answer to the question.
– πάντα ῥεῖ
1 hour ago
You want
nullptr
- always.– Jesper Juhl
1 hour ago
You want
nullptr
- always.– Jesper Juhl
1 hour ago
add a comment |
3 Answers
3
active
oldest
votes
__null
is a g++
internal thing that serves roughly the same purpose as the standard nullptr
added in C++11 (acting consistently as a pointer, never an integer).
NULL
is defined as 0
, which can be implicitly used as integer, boolean, floating point value or pointer, which is a problem when it comes to overload resolution, when you want to call the function that takes a pointer specifically.
In any event, you shouldn't use __null
because it's a g++
implementation detail, so using it guarantees non-portable code. If you can rely on C++11 (surely you can by now?), use nullptr
. If not, NULL
is your only portable option.
2
Note thatNULL
does not have to be defined as0
. It's whatever the implementation chooses as a null-pointer constant.
– Pete Becker
1 hour ago
add a comment |
NULL
is the old C symbol for a null pointer. C++ traditionally have used 0
for null pointers, and since the C++11 standard nullptr
.
Considering that x
doesn't seem to be a pointer then you can't initialize x
to be a null pointer, and the __null
symbol is perhaps some compiler-internal symbol for a null value (which is a concept that doesn't really exist in standard C++).
If you want x
to initialized to some default state, then you have to rely on the MyClass
default constructor to initialize the objects and its member variables to some suitable default values.
add a comment |
NULL
has been overtaken from C into C++ and - prior to C++11 - adopted its C meaning:
until C++11: The macro NULL is an implementation-defined null pointer
constant, which may be an integral constant expression rvalue of
integer type that evaluates to zero.
C++11 then introduced a dedicated null pointer literal nullptr
of type std::nullptr_t
. But - probably for backward compatibility - makro NULL
was not removed; its definition was just a bit relaxed in that compilers may now define it either as integral or as pointer type:
C++11 onwards: an integer literal with value zero, or a prvalue of
type std::nullptr_t
If you use NULL
, then you get implementation-defined behaviour in overload resolution. Consider, for example, the following code with a compiler that uses the integral-version of NULL
-makro. Then a call using NULL
as parameter passed to a function may lead to ambiguities:
struct SomeOverload {
SomeOverload(int x) {
cout << "taking int param: " << x << endl;
}
SomeOverload(void* x) {
cout << "taking void* param: " << x << endl;
}
};
int main() {
int someVal = 10;
SomeOverload a(0);
SomeOverload b(&someVal);
// SomeOverload c(NULL); // Call to constructor is ambiuous
SomeOverload d(nullptr);
}
So it is recommended to use nullptr
where ever you want to express pointer type.
And don't use __null
, as this is a compiler-specific, non-portable constant; nullptr
, in contrast, is perfectly portable.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53963646%2fc-null-vs-null%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
__null
is a g++
internal thing that serves roughly the same purpose as the standard nullptr
added in C++11 (acting consistently as a pointer, never an integer).
NULL
is defined as 0
, which can be implicitly used as integer, boolean, floating point value or pointer, which is a problem when it comes to overload resolution, when you want to call the function that takes a pointer specifically.
In any event, you shouldn't use __null
because it's a g++
implementation detail, so using it guarantees non-portable code. If you can rely on C++11 (surely you can by now?), use nullptr
. If not, NULL
is your only portable option.
2
Note thatNULL
does not have to be defined as0
. It's whatever the implementation chooses as a null-pointer constant.
– Pete Becker
1 hour ago
add a comment |
__null
is a g++
internal thing that serves roughly the same purpose as the standard nullptr
added in C++11 (acting consistently as a pointer, never an integer).
NULL
is defined as 0
, which can be implicitly used as integer, boolean, floating point value or pointer, which is a problem when it comes to overload resolution, when you want to call the function that takes a pointer specifically.
In any event, you shouldn't use __null
because it's a g++
implementation detail, so using it guarantees non-portable code. If you can rely on C++11 (surely you can by now?), use nullptr
. If not, NULL
is your only portable option.
2
Note thatNULL
does not have to be defined as0
. It's whatever the implementation chooses as a null-pointer constant.
– Pete Becker
1 hour ago
add a comment |
__null
is a g++
internal thing that serves roughly the same purpose as the standard nullptr
added in C++11 (acting consistently as a pointer, never an integer).
NULL
is defined as 0
, which can be implicitly used as integer, boolean, floating point value or pointer, which is a problem when it comes to overload resolution, when you want to call the function that takes a pointer specifically.
In any event, you shouldn't use __null
because it's a g++
implementation detail, so using it guarantees non-portable code. If you can rely on C++11 (surely you can by now?), use nullptr
. If not, NULL
is your only portable option.
__null
is a g++
internal thing that serves roughly the same purpose as the standard nullptr
added in C++11 (acting consistently as a pointer, never an integer).
NULL
is defined as 0
, which can be implicitly used as integer, boolean, floating point value or pointer, which is a problem when it comes to overload resolution, when you want to call the function that takes a pointer specifically.
In any event, you shouldn't use __null
because it's a g++
implementation detail, so using it guarantees non-portable code. If you can rely on C++11 (surely you can by now?), use nullptr
. If not, NULL
is your only portable option.
answered 1 hour ago


ShadowRanger
57.1k44993
57.1k44993
2
Note thatNULL
does not have to be defined as0
. It's whatever the implementation chooses as a null-pointer constant.
– Pete Becker
1 hour ago
add a comment |
2
Note thatNULL
does not have to be defined as0
. It's whatever the implementation chooses as a null-pointer constant.
– Pete Becker
1 hour ago
2
2
Note that
NULL
does not have to be defined as 0
. It's whatever the implementation chooses as a null-pointer constant.– Pete Becker
1 hour ago
Note that
NULL
does not have to be defined as 0
. It's whatever the implementation chooses as a null-pointer constant.– Pete Becker
1 hour ago
add a comment |
NULL
is the old C symbol for a null pointer. C++ traditionally have used 0
for null pointers, and since the C++11 standard nullptr
.
Considering that x
doesn't seem to be a pointer then you can't initialize x
to be a null pointer, and the __null
symbol is perhaps some compiler-internal symbol for a null value (which is a concept that doesn't really exist in standard C++).
If you want x
to initialized to some default state, then you have to rely on the MyClass
default constructor to initialize the objects and its member variables to some suitable default values.
add a comment |
NULL
is the old C symbol for a null pointer. C++ traditionally have used 0
for null pointers, and since the C++11 standard nullptr
.
Considering that x
doesn't seem to be a pointer then you can't initialize x
to be a null pointer, and the __null
symbol is perhaps some compiler-internal symbol for a null value (which is a concept that doesn't really exist in standard C++).
If you want x
to initialized to some default state, then you have to rely on the MyClass
default constructor to initialize the objects and its member variables to some suitable default values.
add a comment |
NULL
is the old C symbol for a null pointer. C++ traditionally have used 0
for null pointers, and since the C++11 standard nullptr
.
Considering that x
doesn't seem to be a pointer then you can't initialize x
to be a null pointer, and the __null
symbol is perhaps some compiler-internal symbol for a null value (which is a concept that doesn't really exist in standard C++).
If you want x
to initialized to some default state, then you have to rely on the MyClass
default constructor to initialize the objects and its member variables to some suitable default values.
NULL
is the old C symbol for a null pointer. C++ traditionally have used 0
for null pointers, and since the C++11 standard nullptr
.
Considering that x
doesn't seem to be a pointer then you can't initialize x
to be a null pointer, and the __null
symbol is perhaps some compiler-internal symbol for a null value (which is a concept that doesn't really exist in standard C++).
If you want x
to initialized to some default state, then you have to rely on the MyClass
default constructor to initialize the objects and its member variables to some suitable default values.
answered 1 hour ago


Some programmer dude
294k24248410
294k24248410
add a comment |
add a comment |
NULL
has been overtaken from C into C++ and - prior to C++11 - adopted its C meaning:
until C++11: The macro NULL is an implementation-defined null pointer
constant, which may be an integral constant expression rvalue of
integer type that evaluates to zero.
C++11 then introduced a dedicated null pointer literal nullptr
of type std::nullptr_t
. But - probably for backward compatibility - makro NULL
was not removed; its definition was just a bit relaxed in that compilers may now define it either as integral or as pointer type:
C++11 onwards: an integer literal with value zero, or a prvalue of
type std::nullptr_t
If you use NULL
, then you get implementation-defined behaviour in overload resolution. Consider, for example, the following code with a compiler that uses the integral-version of NULL
-makro. Then a call using NULL
as parameter passed to a function may lead to ambiguities:
struct SomeOverload {
SomeOverload(int x) {
cout << "taking int param: " << x << endl;
}
SomeOverload(void* x) {
cout << "taking void* param: " << x << endl;
}
};
int main() {
int someVal = 10;
SomeOverload a(0);
SomeOverload b(&someVal);
// SomeOverload c(NULL); // Call to constructor is ambiuous
SomeOverload d(nullptr);
}
So it is recommended to use nullptr
where ever you want to express pointer type.
And don't use __null
, as this is a compiler-specific, non-portable constant; nullptr
, in contrast, is perfectly portable.
add a comment |
NULL
has been overtaken from C into C++ and - prior to C++11 - adopted its C meaning:
until C++11: The macro NULL is an implementation-defined null pointer
constant, which may be an integral constant expression rvalue of
integer type that evaluates to zero.
C++11 then introduced a dedicated null pointer literal nullptr
of type std::nullptr_t
. But - probably for backward compatibility - makro NULL
was not removed; its definition was just a bit relaxed in that compilers may now define it either as integral or as pointer type:
C++11 onwards: an integer literal with value zero, or a prvalue of
type std::nullptr_t
If you use NULL
, then you get implementation-defined behaviour in overload resolution. Consider, for example, the following code with a compiler that uses the integral-version of NULL
-makro. Then a call using NULL
as parameter passed to a function may lead to ambiguities:
struct SomeOverload {
SomeOverload(int x) {
cout << "taking int param: " << x << endl;
}
SomeOverload(void* x) {
cout << "taking void* param: " << x << endl;
}
};
int main() {
int someVal = 10;
SomeOverload a(0);
SomeOverload b(&someVal);
// SomeOverload c(NULL); // Call to constructor is ambiuous
SomeOverload d(nullptr);
}
So it is recommended to use nullptr
where ever you want to express pointer type.
And don't use __null
, as this is a compiler-specific, non-portable constant; nullptr
, in contrast, is perfectly portable.
add a comment |
NULL
has been overtaken from C into C++ and - prior to C++11 - adopted its C meaning:
until C++11: The macro NULL is an implementation-defined null pointer
constant, which may be an integral constant expression rvalue of
integer type that evaluates to zero.
C++11 then introduced a dedicated null pointer literal nullptr
of type std::nullptr_t
. But - probably for backward compatibility - makro NULL
was not removed; its definition was just a bit relaxed in that compilers may now define it either as integral or as pointer type:
C++11 onwards: an integer literal with value zero, or a prvalue of
type std::nullptr_t
If you use NULL
, then you get implementation-defined behaviour in overload resolution. Consider, for example, the following code with a compiler that uses the integral-version of NULL
-makro. Then a call using NULL
as parameter passed to a function may lead to ambiguities:
struct SomeOverload {
SomeOverload(int x) {
cout << "taking int param: " << x << endl;
}
SomeOverload(void* x) {
cout << "taking void* param: " << x << endl;
}
};
int main() {
int someVal = 10;
SomeOverload a(0);
SomeOverload b(&someVal);
// SomeOverload c(NULL); // Call to constructor is ambiuous
SomeOverload d(nullptr);
}
So it is recommended to use nullptr
where ever you want to express pointer type.
And don't use __null
, as this is a compiler-specific, non-portable constant; nullptr
, in contrast, is perfectly portable.
NULL
has been overtaken from C into C++ and - prior to C++11 - adopted its C meaning:
until C++11: The macro NULL is an implementation-defined null pointer
constant, which may be an integral constant expression rvalue of
integer type that evaluates to zero.
C++11 then introduced a dedicated null pointer literal nullptr
of type std::nullptr_t
. But - probably for backward compatibility - makro NULL
was not removed; its definition was just a bit relaxed in that compilers may now define it either as integral or as pointer type:
C++11 onwards: an integer literal with value zero, or a prvalue of
type std::nullptr_t
If you use NULL
, then you get implementation-defined behaviour in overload resolution. Consider, for example, the following code with a compiler that uses the integral-version of NULL
-makro. Then a call using NULL
as parameter passed to a function may lead to ambiguities:
struct SomeOverload {
SomeOverload(int x) {
cout << "taking int param: " << x << endl;
}
SomeOverload(void* x) {
cout << "taking void* param: " << x << endl;
}
};
int main() {
int someVal = 10;
SomeOverload a(0);
SomeOverload b(&someVal);
// SomeOverload c(NULL); // Call to constructor is ambiuous
SomeOverload d(nullptr);
}
So it is recommended to use nullptr
where ever you want to express pointer type.
And don't use __null
, as this is a compiler-specific, non-portable constant; nullptr
, in contrast, is perfectly portable.
answered 1 hour ago


Stephan Lechner
25.5k21839
25.5k21839
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53963646%2fc-null-vs-null%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
psL SDkbMfr8iXFEyntroes339l7jqJ08xA23,wNyy LG4efbZFzw,46dRlG,Clm 0g85 vIL,0
14
In C++11 and after you should use the keyword nullptr.
– Anon Mail
1 hour ago
1
Don't use
__null
. If it's an implementation detail, it's not portable to use it. If it's defined by the project, it's using a name reserved for use by the implementation, it's not legal to use that identifier and it should be removed from the project.– François Andrieux
1 hour ago
If you want
NULL
usenullptr
. If that doesn't compile then you don't have a pointer and you are doing the wrong thing.– NathanOliver
1 hour ago
@Some That's probably an appropriate answer to the question.
– πάντα ῥεῖ
1 hour ago
You want
nullptr
- always.– Jesper Juhl
1 hour ago